Unlike the bound grid (the BoundGridControl class), the unbound grid (the UnboundGridControl class) is not bound to a particular data source and should be populated with data manually. By using UnboundGridControl you have absolute control over the grid content by adding, removing, and modifying rows, cells and other grid elements. Built-in tree grid-like functionality with nested group rows and data rows allows you to group and display data in a logical and structured way.
There are two types of rows in the UnboundGridControl class: GroupRow and DataRow . The first actually contains no data and allows you to form logical groups consisting of group and data rows. The second contains cells with data.
You can create a new group row with the UnboundGridControl.NewGroupRow method. The group row's property GroupRow.Rows represents a collection of its child group and data rows. So you can use methods of the RowCollection class to control child rows of any group row.
The UnboundGridControl.NewDataRow method returns a new DataRow object. This method has two overloads. The first overload takes no arguments and the second takes a data source object for whole row. After you have a row, you can add cells to it by using its DataRow.AddNewCell method.
The following example shows how to manually initialize a grid with cells and data:
[C#]
using Elegant.Grid;
private void Form1_Load(object sender, System.EventArgs e)
{
Column column1 = new Column();
column1.HeaderText = "Column 1";
Column column2 = new Column();
column2.HeaderText = "Column 2";
Column column3 = new Column();
column3.HeaderText = "Column 3";
unboundGridControl1.Columns.AddRange(
new Column[]
{
column1,
column2,
column3
});
unboundGridControl1.ColumnsAutoSizeMode = ColumnAutoSizeMode.Fill;
DataRow dataRow1 = unboundGridControl1.NewDataRow();
TextCell textCell1 =
dataRow1.AddNewCell(typeof(TextCell), column1) as TextCell;
textCell1.DataValue = "Some text...";
textCell1.Style.TextAlignment = ContentAlignment.MiddleCenter;
CheckBoxCell CheckBoxCell1 =
dataRow1.AddNewCell(typeof(CheckBoxCell), column2) as CheckBoxCell;
CheckBoxCell1.DataValue = true;
DateTimeCell progressBarCell1 =
dataRow1.AddNewCell(typeof(DateTimeCell), column3) as DateTimeCell;
progressBarCell1.DataValue = DateTime.Now;
progressBarCell1.Style.TextAlignment = ContentAlignment.MiddleCenter;
unboundGridControl1.Rows.Add(dataRow1);
DataRow dataRow2 = unboundGridControl1.NewDataRow();
ColorCell colorCell2 = dataRow2.AddNewCell(typeof(ColorCell), column1) as
ColorCell;
colorCell2.DataValue = Color.LightPink;
TextCell textCell2 = dataRow2.AddNewCell(typeof(TextCell), column2) as
TextCell;
textCell2.DataValue = "Some text...";
textCell2.Style.TextAlignment = ContentAlignment.MiddleCenter;
ProgressBarCell progressBarCell2 =
dataRow2.AddNewCell(typeof(ProgressBarCell), column3) as
ProgressBarCell;
progressBarCell2.DataValue = 85;
progressBarCell2.Style.ProgressBarColor1 = Color.Red;
progressBarCell2.Style.ProgressBarColor2 = Color.LightSkyBlue;
GroupRow groupRow1 = unboundGridControl1.NewGroupRow();
groupRow1.Text = "Group row 1";
groupRow1.Rows.AddRange(new Row[]{dataRow2});
unboundGridControl1.Rows.Add(groupRow1);
DataRow dataRow3 = unboundGridControl1.NewDataRow();
ProgressBarCell progressBarCell3 =
dataRow3.AddNewCell(typeof(ProgressBarCell), column1) as
ProgressBarCell;
progressBarCell3.DataValue = 90;
progressBarCell3.Style.ProgressBarBrushType =
ProgressBarBrushType.PathGradient;
progressBarCell3.Style.ProgressBarColor1 = Color.Red;
progressBarCell3.Style.ProgressBarColor2 = Color.LightSkyBlue;
progressBarCell3.Style.ProgressBarPathGradientCenterColor = Color.White;
NumericCell textCell3 =
dataRow3.AddNewCell(typeof(NumericCell), column2) as NumericCell;
textCell3.DataValue = 60;
textCell3.Style.TextAlignment = ContentAlignment.MiddleCenter;
ColorCell colorCell3 =
dataRow3.AddNewCell(typeof(ColorCell), column3) as ColorCell;
colorCell3.DataValue = Color.Coral;
GroupRow groupRow2 = unboundGridControl1.NewGroupRow();
groupRow2.Text = "Group row 2";
GroupRow groupRow3 = unboundGridControl1.NewGroupRow();
groupRow3.Text = "Group row 3";
groupRow3.Rows.AddRange(new Row[]{dataRow3});
groupRow2.Rows.AddRange(new Row[]{groupRow3});
unboundGridControl1.Rows.Add(groupRow2);
}
[VB.NET]
Imports Elegant.Grid
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As _
System.EventArgs) Handles MyBase.Load
Dim column1 As New Column
column1.HeaderText = "Column 1"
Dim column2 As New Column
column2.HeaderText = "Column 2"
Dim column3 As New Column
column3.HeaderText = "Column 3"
unboundGridControl1.Columns.AddRange( _
new Column() _
{ _
column1, _
column2, _
column3 _
})
UnboundGridControl1.ColumnsAutoSizeMode = ColumnAutoSizeMode.Fill
Dim dataRow1 = UnboundGridControl1.NewDataRow()
Dim textCell1 As TextCell = dataRow1.AddNewCell( _
GetType(TextCell), column1)
textCell1.DataValue = "Some text..."
textCell1.Style.TextAlignment = ContentAlignment.MiddleCenter
Dim CheckBoxCell1 As CheckBoxCell = _
dataRow1.AddNewCell(GetType(CheckBoxCell), column2)
CheckBoxCell1.DataValue = True
Dim progressBarCell1 As DateTimeCell = _
dataRow1.AddNewCell(GetType(DateTimeCell), column3)
progressBarCell1.DataValue = DateTime.Now
progressBarCell1.Style.TextAlignment = ContentAlignment.MiddleCenter
UnboundGridControl1.Rows.Add(dataRow1)
Dim dataRow2 = UnboundGridControl1.NewDataRow()
Dim colorCell2 As ColorCell = dataRow2.AddNewCell( _
GetType(ColorCell), column1)
colorCell2.DataValue = Color.LightPink
Dim textCell2 As TextCell = dataRow2.AddNewCell( _
GetType(TextCell), column2)
textCell2.DataValue = "Some text..."
textCell2.Style.TextAlignment = ContentAlignment.MiddleCenter
Dim progressBarCell2 As ProgressBarCell = _
dataRow2.AddNewCell(GetType(ProgressBarCell), column3)
progressBarCell2.DataValue = 85
progressBarCell2.Style.ProgressBarColor1 = Color.Red
progressBarCell2.Style.ProgressBarColor2 = Color.LightSkyBlue
Dim groupRow1 = UnboundGridControl1.NewGroupRow()
groupRow1.Text = "Group row 1"
groupRow1.Rows.AddRange(New Row() {dataRow2})
UnboundGridControl1.Rows.Add(groupRow1)
Dim dataRow3 = UnboundGridControl1.NewDataRow()
Dim progressBarCell3 As ProgressBarCell = _
dataRow3.AddNewCell(GetType(ProgressBarCell), column1)
progressBarCell3.DataValue = 90
progressBarCell3.Style.ProgressBarBrushType =
ProgressBarBrushType.PathGradient
progressBarCell3.Style.ProgressBarColor1 = Color.Red
progressBarCell3.Style.ProgressBarColor2 = Color.LightSkyBlue
progressBarCell3.Style.ProgressBarPathGradientCenterColor = Color.White
Dim textCell3 As NumericCell = dataRow3.AddNewCell( _
GetType(NumericCell), column2)
textCell3.DataValue = 60
textCell3.Style.TextAlignment = ContentAlignment.MiddleCenter
Dim colorCell3 As ColorCell = dataRow3.AddNewCell( _
GetType(ColorCell), column3)
colorCell3.DataValue = Color.Coral
Dim groupRow2 = UnboundGridControl1.NewGroupRow()
groupRow2.Text = "Group row 2"
Dim groupRow3 = UnboundGridControl1.NewGroupRow()
groupRow3.Text = "Group row 3"
groupRow3.Rows.AddRange(New Row() {dataRow2})
groupRow2.Rows.AddRange(New Row() {groupRow3})
UnboundGridControl1.Rows.Add(groupRow2)
End Sub
After the above code has been executed the grid will look similar to the one displayed below:
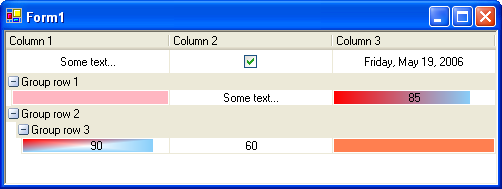
Figure 1 Unbound grid with group rows and cells of non-equal types in columns
The UnboundGridControl.AutoCreateCells property set to true allows you to create all the row cells automatically when a new data row is created with the UnboundGridControl.NewDataRow method. But in this case you should create all the columns before creating any data row. The type of each created cell is defined by the column's property Column.CellType . The following example demonstrates how to initialize a grid in a simplified way using automatic cells creation and the UnboundGridControl.NewDataRow(object data) method:
[C#]
using Elegant.Grid;
private void Form1_Load(object sender, System.EventArgs e)
{
Column firstNameColumn = new Column();
firstNameColumn.HeaderText = "First Name";
firstNameColumn.DataPropertyName = "[0]";
Column lastNameColumn = new Column();
lastNameColumn.HeaderText = "Last Name";
lastNameColumn.DataPropertyName = "[1]";
Column birthDateColumn = new Column();
birthDateColumn.HeaderText = "Birth Date";
birthDateColumn.DataPropertyName = "[2]";
birthDateColumn.CellType = typeof(DateTimeCell);
unboundGridControl1.Columns.AddRange(
new Column[]
{
firstNameColumn,
lastNameColumn,
birthDateColumn
});
unboundGridControl1.ColumnsAutoSizeMode = ColumnAutoSizeMode.Fill;
unboundGridControl1.AutoCreateCells = true;
object[][] dataSource = new object[5][]
{
new object[]{"Catherine", "Abel", new DateTime(1975, 10, 20)},
new object[]{"Kim", "Abercrombie", new DateTime(1960, 8, 17)},
new object[]{"Humberto", "Acevedo", new DateTime(1971, 3, 27)},
new object[]{"Gustavo", "Achong", new DateTime(1980, 2, 23)},
new object[]{"Carla", "Adams", new DateTime(1958, 11, 5)}
};
unboundGridControl1.Rows.AddRange(
new Row[]
{
unboundGridControl1.NewDataRow(dataSource[0]),
unboundGridControl1.NewDataRow(dataSource[1]),
unboundGridControl1.NewDataRow(dataSource[2]),
unboundGridControl1.NewDataRow(dataSource[3]),
unboundGridControl1.NewDataRow(dataSource[4])
});
}
[VB.NET]
Imports Elegant.Grid
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As _
System.EventArgs) Handles MyBase.Load
Dim column1 As New Column
Dim firstNameColumn As New Column
firstNameColumn.HeaderText = "First Name"
firstNameColumn.DataPropertyName = "[0]"
Dim lastNameColumn As New Column
lastNameColumn.HeaderText = "Last Name"
lastNameColumn.DataPropertyName = "[1]"
Dim birthDateColumn As New Column
birthDateColumn.HeaderText = "Birth Date"
birthDateColumn.DataPropertyName = "[2]"
birthDateColumn.CellType = GetType(DateTimeCell)
unboundGridControl1.Columns.AddRange( _
New Column() _
{ _
firstNameColumn, _
lastNameColumn, _
birthDateColumn _
})
UnboundGridControl1.ColumnsAutoSizeMode = ColumnAutoSizeMode.Fill
UnboundGridControl1.AutoCreateCells = True
Dim dataSource()() As Object = _
{ _
New Object() {"Catherine", "Abel", New DateTime(1975, 10, 20)}, _
New Object() {"Kim", "Abercrombie", New DateTime(1960, 8, 17)}, _
New Object() {"Humberto", "Acevedo", New DateTime(1971, 3, 27)}, _
New Object() {"Gustavo", "Achong", New DateTime(1980, 2, 23)}, _
New Object() {"Carla", "Adams", New DateTime(1958, 11, 5)} _
}
UnboundGridControl1.Rows.AddRange( _
New Row() _
{ _
UnboundGridControl1.NewDataRow(dataSource(0)), _
UnboundGridControl1.NewDataRow(dataSource(1)), _
UnboundGridControl1.NewDataRow(dataSource(2)), _
UnboundGridControl1.NewDataRow(dataSource(3)), _
UnboundGridControl1.NewDataRow(dataSource(4)) _
})
End Sub
The code above produces the grid shows on the Figure 2.
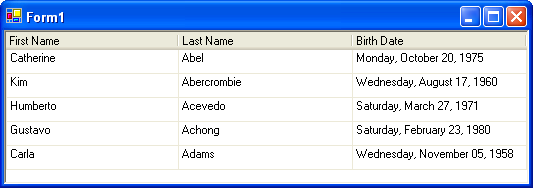
Figure 2 Unbound grid with each column having cells of the same type
If you decide to create cells automatically (UnboundGridControl.AutoCreateCells == true ) and for some reason you need to make one or more cells in the same column having a different type than that specified in Column.CellType , you can always do this in the handler of the CreateCell event.
|