If the built-in cell editor for a particular cell type is not completely what you are looking for, or if a particular cell type does not feature any cell editor at all, you can implement your own editor by creating a class that implements the ICellEditor interface:
Member
|
Description
|
Activate method |
Invoked when the editor is activated. The method takes one argument which represents the instance of the edited cell.
|
Deactivate method |
Invoked when editing is complete. You can use this method to do some finalizing operations. |
Value property |
Gets or sets the current value of the editor. |
ValueChanged event |
Occurs when the current value of the editor changes. You can raise this event in your editor to process data changes with the GridControlBase.ValidateData event in the calling code. |
The Elegant Grid knows nothing about the details of the custom editor's implementation, so it is your responsibility to initialize the editor in the ICellEditor.Activate method and destroy it in ICellEditor.Deactivate .
The ICellEditor.Activate method takes one argument of the Cell type, which allows you to get all the information about the edited cell: its layout, a reference to the parent grid object and other cell properties.
The Cell.CommitEdit method allows you to save the changes made to the editor's content. The method takes a single parameter which specifies whether the editor should be closed. To close the editor without saving, call Cell.CancelEdit .
The last thing which deserves to be mentioned here is how to specify the key with which the editor is activated. It is really simple: just use the ActivateByKey attribute class before your editor call declaration. You can specify one or more ActivateByKey attributes for your editor.
The following example illustrates how to implement a custom editor for cells of the ProgressBarCell type.
[C#]
using Elegant.Grid;
[ActivateByKey(Keys.F2)]
[ActivateByKey(Keys.F10)]
[ActivateByKey(Keys.Enter)]
public class ProgressCellCustomEditor : TrackBar, ICellEditor
{
public ProgressCellCustomEditor()
{
Minimum = 0;
Maximum = 100;
AutoSize = false;
Hide();
}
public void Activate(Cell cell)
{
_EditingCell = cell as ProgressBarCell;
if(_EditingCell == null)
return;
Parent = cell.GridControl;
BackColor = _EditingCell.InheritedStyle.ProgressBarColor1;
Bounds = _EditingCell.ContentRectangle;
TickStyle = TickStyle.None;
Show();
Focus();
}
public void Deactivate()
{
Hide();
_EditingCell = null;
}
private ProgressBarCell _EditingCell;
object ICellEditor.Value
{
get
{
return base.Value;
}
set
{
base.Value = Convert.ToInt32(value);
}
}
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if(_EditingCell == null)
return;
if(e.KeyCode == Keys.Escape)
{
_EditingCell.CancelEdit();
}
else if(e.KeyCode == Keys.Enter)
{
_EditingCell.CommitEdit (true);
}
}
protected override void OnLostFocus(EventArgs e)
{
base.OnLostFocus(e);
if(_EditingCell != null)
_EditingCell.CancelEdit();
}
}
[VB.NET]
Imports Elegant.Grid
< _
ActivateByKeyAttribute(Keys.F2), _
ActivateByKey(Keys.F10), _
ActivateByKey(Keys.Enter) _
> _
Public Class ProgressCellCustomEditor
Inherits TrackBar
Implements ICellEditor
Public Sub New()
Minimum = 0
Maximum = 100
AutoSize = False
Hide()
End Sub
Public Sub Activate(ByVal cell As Cell) Implements ICellEditor.Activate
_EditingCell = cell
If (_EditingCell Is Nothing) Then
Return
End If
Parent = cell.GridControl
BackColor = _EditingCell.InheritedStyle.ProgressBarColor1
Bounds = _EditingCell.ContentRectangle
TickStyle = TickStyle.None
Show()
Focus()
End Sub
Public Sub Deactivate() Implements ICellEditor.Deactivate
Hide()
_EditingCell = Nothing
End Sub
Private _EditingCell As ProgressBarCell
Public Shadows Property Value() As Object Implements ICellEditor.Value
Get
Return MyBase.Value
End Get
Set(ByVal Value)
MyBase.Value = Convert.ToInt32(Value)
End Set
End Property
Public Shadows Event ValueChanged(ByVal sender As Object, ByVal e As _
EventArgs) Implements ICellEditor.ValueChanged
Protected Overridable Sub OnTrackBarValueChanged(ByVal sender As _
Object, ByVal e As EventArgs) Handles MyBase.ValueChanged
RaiseEvent ValueChanged(Me, EventArgs.Empty)
End Sub
Protected Overrides Sub OnKeyDown(ByVal e As KeyEventArgs)
MyBase.OnKeyDown(e)
If (_EditingCell Is Nothing) Then
Return
End If
If (e.KeyCode = Keys.Escape) Then
_EditingCell.CancelEdit()
ElseIf (e.KeyCode = Keys.Enter) Then
_EditingCell.CommitEdit(True)
End If
End Sub
Protected Overloads Sub OnLostFocus(ByVal e As EventArgs)
MyBase.OnLostFocus(e)
If (Not (_EditingCell Is Nothing)) Then
_EditingCell.CancelEdit()
End If
End Sub
End Class
Here is how to use this custom editor in a grid.
[C#]
using Elegant.Grid;
private void Form1_Load(object sender, System.EventArgs e)
{
Column nameColumn = new Column();
nameColumn.HeaderText = "Name";
nameColumn.DataPropertyName = "[0]";
Column taskColumn = new Column();
taskColumn.HeaderText = "Task Name";
taskColumn.DataPropertyName = "[1]";
Column completitionStatusColumn = new Column();
completitionStatusColumn.HeaderText = "Completion Status";
completitionStatusColumn.DataPropertyName = "[2]";
completitionStatusColumn.CellType = typeof(ProgressBarCell);
boundGridControl1.Columns.AddRange(
new Column[]
{
nameColumn,
taskColumn,
completitionStatusColumn
}
);
boundGridControl1.ColumnsAutoSizeMode = ColumnAutoSizeMode.Fill;
//
// Data source initialization here...
//
boundGridControl1.DataSource = dataSource;
}
[VB.NET]
Imports Elegant.Grid
Private Sub Form1_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles MyBase.Load
Dim nameColumn As New Column
nameColumn.HeaderText = "Name"
nameColumn.DataPropertyName = "[0]"
Dim taskColumn As New Column
taskColumn.HeaderText = "Task Name"
taskColumn.DataPropertyName = "[1]"
Dim completitionStatusColumn As New Column
completitionStatusColumn.HeaderText = "Completion Status"
completitionStatusColumn.DataPropertyName = "[2]"
completitionStatusColumn.CellType = GetType(ProgressBarCell)
completitionStatusColumn.CellStyles( _
GetType(ProgressBarCellStyle)).CustomEditorType = _
GetType(ProgressCellCustomEditor)
BoundGridControl1.Columns.AddRange(New Column() { _
nameColumn, taskColumn, completitionStatusColumn})
BoundGridControl1.ColumnsAutoSizeMode = ColumnAutoSizeMode.Fill
'Data source initialization goes here...
BoundGridControl1.DataSource = dataSource
End Sub
The implemented custom editor is shown on Figure 1. 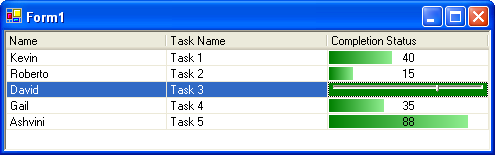
Figure 1 Custom editor for the progress bar cell
|